Getting Started
You can import sty like this:
import sty
However, if you need to style a lot of stuff, you might consider importing the register-objects directly, like this:
from sty import fg, bg, ef, rs
Sty all the strings!
from sty import bg, ef, fg, rs
foo = fg.red + "This is red text!" + fg.rs
bar = bg.blue + "This has a blue background!" + bg.rs
baz = ef.italic + "This is italic text" + rs.italic
qux = fg(201) + "This is pink text using 8bit colors" + fg.rs
qui = fg(255, 10, 10) + "This is red text using 24bit colors." + fg.rs
# Add custom colors:
from sty import RgbFg, Style
fg.orange = Style(RgbFg(255, 150, 50))
buf = fg.orange + "Yay, Im orange." + fg.rs
print(foo, bar, baz, qux, qui, buf, sep="\n")
The code above will print like this in the terminal:
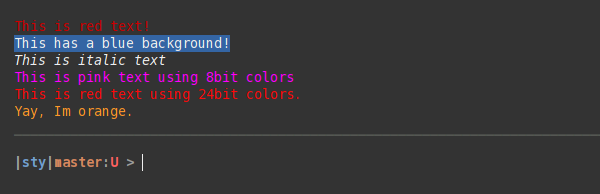
A quick look at the primitives:
sty provides a bunch of tiny, but flexible primitives (called register-objects) that can be used to style your strings:
ef
(effect-register)fg
(foreground-register)bg
(background-register)rs
(reset-register)
Each register-object carries a default selection of attributes, which you can select like this:
ef.italic
fg.blue
bg.green
rs.all
Or like this, which is nice in case you need to dynamically select attributes:
a = ef("italic") + "Italic text." + rs.italic
b = fg("blue") + "Blue text." + rs.fg
c = bg(randint(0, 254)) + "Random colored bg" + rs.bg
print(a, b, c, sep="\n")
fg
and bg
are special in the way that they allow you to select
8bit and 24bit colors directly:
# select an 8bit color directly.
a = fg(196) + "This is red text" + rs.fg
# select a 24bit rgb color directly.
b = bg(50, 255, 50) + "Text with green bg" + rs.bg
print(a, b, sep="\n")
I think this is all you need to know to get going. Check out the documentation or the codebase for more detail or feel free to create an issue and ask. Have fun! :D